Introduction
Sending the user an email with guidelines on connecting before their Cloud PC is ready could lead the user to try to connect before the Cloud PC is ready. Wouldn’t it be cool if a user only gets an email when their Cloud PC is provisioned and ready to use? in my mind, that is the optimal way of giving the information. I will go through the aspect of how this is working.
How to enable this feature
This isn’t a built-in feature in the Windows 365 Service, so we must use Microsoft Graph to accomplish a solution like this. This solution is based on the Microsoft Graph PowerShell SDK module because dealing with PowerShell is much simpler from my point of view.
Before running the script and sending emails to all users with a Cloud PC, it’s essential to understand some important aspects of the script.
How the script works
It’s not that complicated how the script works. First, it will get all the Cloud PC that is done provisioning. From there, it will look at each Cloud PC Azure AD object and check for a specific extension attribute and value. If the value isn’t there, it will send an email to the primary user of the Cloud PC. At last, it will write the value to the extension attribute so the next time the script runs, it knows the user has an email for that Cloud PC device.
The admin user you execute the script with must have a mailbox as the email will be sent from that user. This is something that will be changed in the upcoming updates.
Modules and API Permissions
The script uses Microsoft Graph PowerShell SDK modules to read and write information. All the required PowerShell modules will automatically be installed once you run the script.
The account you authenticate with has to have the following API permission:
- CloudPC.Read.All
- User.Read.All
- Directory.ReadWrite.All
- Mail.Send
- Device.Read.All
- Directory.AccessAsUser.All
Email content and attachment
The email content and attachment that will be sent must be prepared before running the script.
Only HTML format is currently supported for the email content.
The attachment can be any file type. The max size for an attachment is 4 MB, and only one attachment is currently allowed.
Existing Cloud PC devices
As described earlier, the script looks at a specific extension attribute on the AzureAD object. If you already have some users working on their Cloud PC, it might be a good idea to ensure the correct value is present on the existing AzureAD objects so those users won’t get an email.
Fill out the variables in the script below to set it on all your existing Cloud PC devices. Please note that it has to be the same extension attribute and value you use when executing the script for new Cloud PC Devices.
Script execution and result
The script has five parameters to define, all shortly described below. You will find the script with screenshots and results below. In the future, visit my Github to get the latest version of all the scripts, as the scripts in this article won’t be updated.
$ExstensionAttributeKey = Specify which extension attribute the script will use to detect if the email has been sent.
$ExstensionAttributeValue = The value that must exist in the extension attribute.
$MailContentPath = Path to your HTML file that contains your email message.
$EmailAttachment = Path to attachment file. Leave blank if no attachment should be sent.
$EmailSubject = The subject header in the email that is being sent.
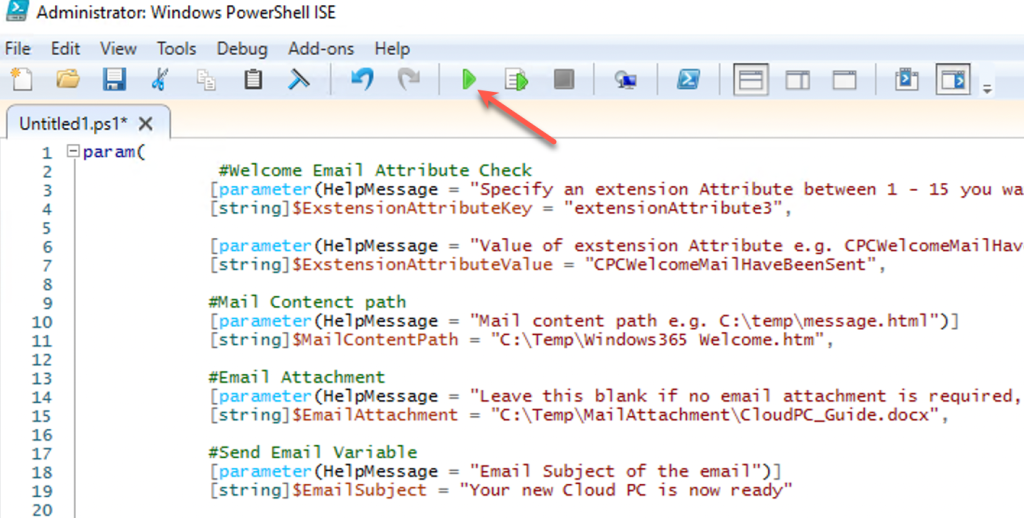
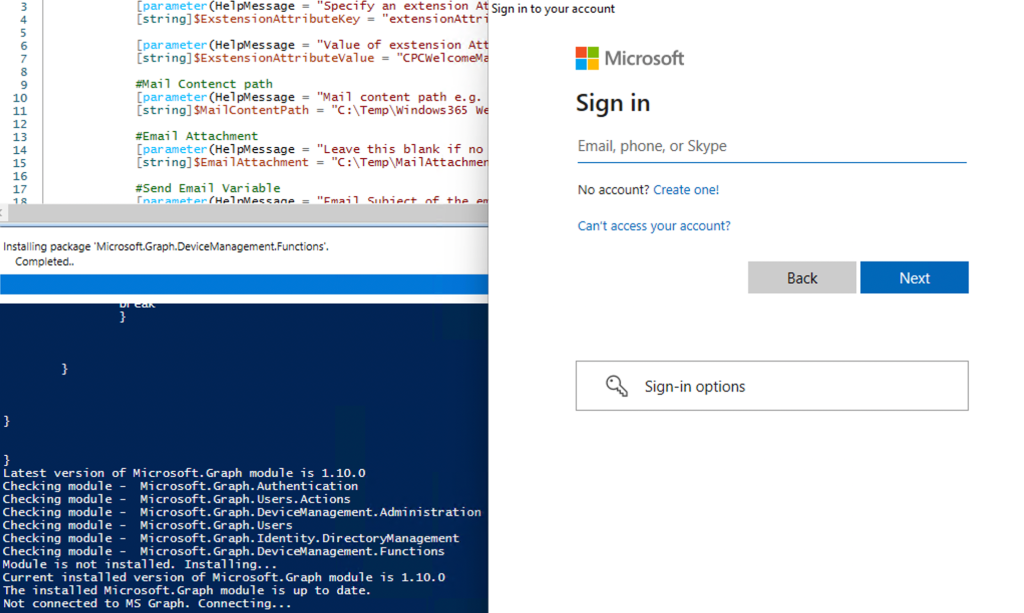

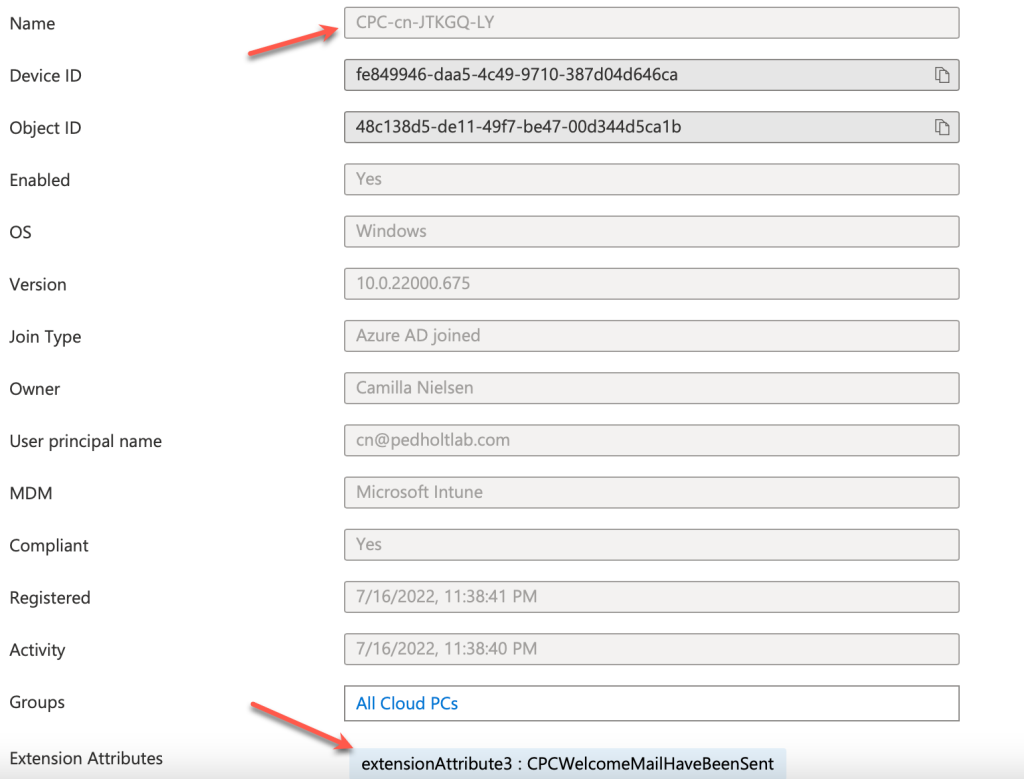
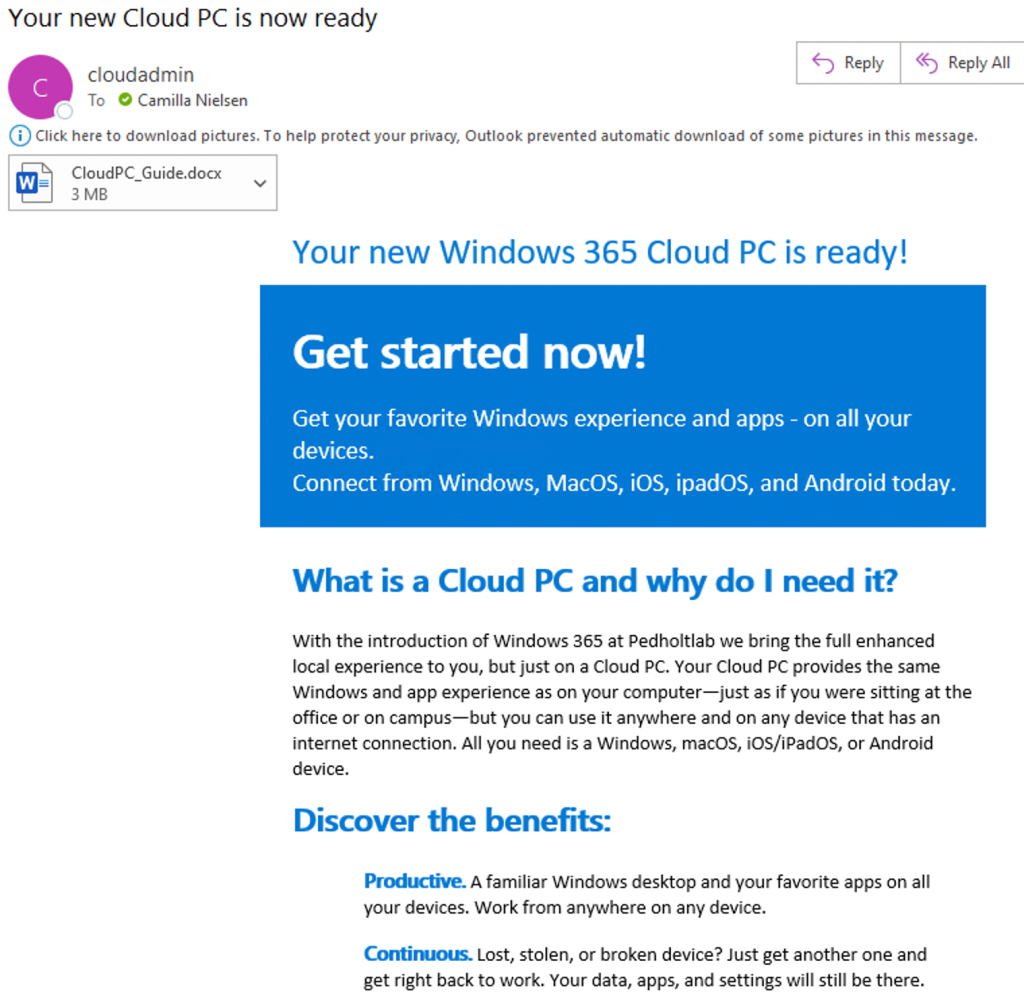
Create an automated flow
Having to run the script manually is not optimal. Therefore it is always a good idea to automate tasks like these. The script must be modified to run in an automated task on a server. With the proper knowledge, it can be done. But wouldn’t it be cool if this could be automated with modern services in Microsoft Azure?
I’m working with my good friend Michael Mardahl on a cloud-based solution in Microsoft Azure that can support this automated flow with Windows 365. Keep an eye on the W365community Youtube Channel or follow us on social media to get updated once it is ready.
Final Thoughts
Even though the current solution has to be executed in hand, sending out information to the user post-provisioning of their Cloud PC may remove some confusion and helpdesk requests. It may also simplify the current communication flow from the IT department. I’m looking forward to sharing an automated solution with Michael Mardahl soon.
Hi Morten,
Thanks for this great article! I’m trying to create a Power Automate Flow to do this very action for new Cloud PC’s deployed to users, so i’m looking forward to seeing the automation you’re working on. Quick question, would you be able to share the HTML e-mail you created?
Thanks